
VivJson is the embedded scripting language and the extension of JSON.
The base concept is that the block {...}
is function.
After evaluating it, it becomes key-value pairs (a.k.a. map, dict, hashes, associative arrays) of host language. Finally, it can be exported as JSON's object.
- Deserialize/serialize JSON.
- Tiny language
- The embedded scripting language
- Dynamically typing
- Lightweight language
- The extension of JSON (JSON's object is valid statements as script.)
JSON offers flexible data structure. Its manipulation is so easy in
dynamically typed language. On the other hand, in statically typed
language, such as Java, it is so difficult.
Thus, this embedded script empowers to manipulate JSON in statically
typed language.
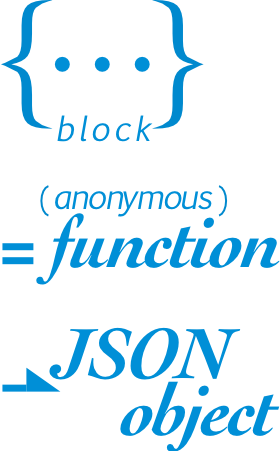
Sample code
{
a = 100
b = 5
b *= 3
function add(x, y) {
return(x + y)
}
data = add(a, b)
}
The result of VivJson is JSON's object.
For example, the following object is the above sample's result.
{
"a": 100,
"b": 15,
"data": 115
}
In VivJson, the explicit statement terminator is not needed.
However it can specify with ;
, ,
, or line-break (new line code).
Thus, {a = 100 b = 5}
, {a = 100; b = 5;}
, and {a = 100, b = 5}
are equivalent.
:
can be used instead of =
for the assignment.
(Note that the variable of the left-hand side is treated as local variable when :
is used.)
The variable of the left-hand side can be enclosed with quotation marks.
As a result, JSON's object is valid statements for VivJson.
x = {"a": 100, "b": 15, "data": 115}
y = {
a = 100
b = 15
data = 115
}
if (x == y) { print('same')}
The unnecessary members (variables) can be removed from the result with using "_" as their prefix.
a
and b
of the above sample are renamed to _a
and _b
as below.
Then its result is {"data": 115}
.
{
_a = 100
_b = 5
_b *= 3
function add(x, y) {
return(x + y)
}
data = add(_a, _b)
}
The outermost curly brackets can be omitted. So the following code is equivalent to the above one.
(Note that this is syntax sugar.)
The result is also {"data": 115}
.
_a = 100
_b = 5
_b *= 3
function add(x, y) {
return(x + y)
}
data = add(_a, _b)
See further samples.
In command line
Manipulating JSON's value is possible as below.
$ python3 -m vivjson '{"foods": [{"name": "orange", "number": 5}, {"name": "fish", "number": 2}]}' 'for(food in foods){print(food.name + ": " + food.number)} return("")'
orange: 5
fish: 2
$ python3 -m vivjson 'a = {"0": [10, 30], "1": 50}, a.0.1 += 80'
{"a": {"0": [10, 110], "1": 50}}
$ python3 -m vivjson 10 20 "return(_[0] + _[1])"
30
$ python3 -m vivjson 5 "return(_ * 2)"
10
$ echo '{"a":3}' | python3 -m vivjson -i 'return(a*2)'
6
Note that JSON's value is not only object but also boolean, null, number, string, and array.
(See RFC 8259 Section 2 and RFC 8259 Section 3.)
The other samples are available:
Use cases
- Change the behavior of Application with downloaded script.
- Change the operation of data with embedded script within data.
- Manipulate JSON's object with script in command line.
Implementation
License
Licensed under the Apache License, Version 2.0.
Alternative
Your wanted solution may be not this script.
The following WEB sites are useful for you.
Personally, we like Lua.
There are the extension of JSON:
JsonLogic can implement logic into standard JSON.
jq can manipulate JSON like sed.
JSONata and Jayway JsonPath can manipulate JSON like XPath.
Reference
Special thanks to the following WEB sites.
They give helpful information for develop.